Spring has just celebrated its 20th anniversary in late 2022, and the Java framework isn’t showing signs of waning popularity. In fact, on our job board, we have hundreds of Spring jobs available for remote developers at any given time.
Anyway, if you made it here, you probably have a Spring interview coming up —
As a hiring manager, what are some of the common and most up-to-date Spring Framework interview questions to ask?
In the guide below, we’ll walk you through Spring interview questions and answers, from basic ones on the fundamentals to tougher questions on aspect-oriented programming, model-view-controller, JDBC, and more. After each of these popular interview questions on Spring, we’ll explain what you should look for in your candidate’s answers.
Let’s get started, shall we?
Looking to hire the best remote developers? Explore HireAI to see how you can:
⚡️ Get instant candidate matches without searching
⚡️ Identify top applicants from our network of 300,000+ devs with no manual screening
⚡️ Hire 4x faster with vetted candidates (qualified and interview-ready)
Try HireAI and hire top developers now →
Spring, Spring Core, and Spring IoC Interview Questions
What is a Spring configuration file?
A Spring configuration file defines the relationship between different classes in the Spring application.
As an interviewer asking this question about the Spring configuration file, you should know that it can be defined differently. Essentially, it defines application behavior by allowing you to declare configurations such as Beans and Bean life cycles.
While the file is traditionally defined as an XML file, these can also be defined as @Configuration
annotations through Spring Core. The annotation method is a modern way of defining Spring configurations and is becoming more commonplace than the XML file. Therefore, it’s important to recognize that questions about the @Configuration
annotation are very similar to questions about the Spring configuration file.
What is the Spring IoC (Inversion of Control) Container?
The Spring IoC Container enables dependency injection by managing bean instantiation and configuration. These are commonly defined with ApplicationContext
or BeanFactory
. Since these serve as a centralized interface for application configuration, every project typically begins with a boilerplate setup of the ApplicationContext
.
IoC is a software principle that enables us to have a loose coupling between objects. In the Spring ecosystem, IoC is displayed when Spring creates an object and provides that object additional metadata and dependencies on its behalf. This is essentially a dependency injection. When you ask your candidate about Spring IoC, they should know that it is essentially a dependency injection in action.
How do you use dependency injection with Spring?
Dependency injection can be achieved in Spring through XML configurations, Spring annotations such as @Autowire
, and configuring the IoC container through ApplicationContext
. By using dependency injection, you can make one service available in another without having to explicitly initialize one since Spring will manage its life cycle.
While @Autowire
is commonly used for setting up dependency injection, Spring 4.3+ will actually scan your classes to manage them on your behalf. This question is perfect if you want to gauge whether your candidate’s familiar with newer versions of Spring!
What is the difference between constructor injection and setter injection?
You may ask your candidates about these types of injections to gauge their depth of understanding regarding Spring dependency injection.
Setter injection defines dependencies after an object is instantiated. Constructor injection defines dependencies when an object is created. In practice, setter injection is typically used for optional dependencies, and constructor injection is used for mandatory dependencies that are required for expected behavior.
To follow up, you may ask your candidate to provide an example of how they may have used setter or constructor injection in object definitions. This helps you better understand whether their understanding of Spring dependency injections is mostly theoretical or backed by hands-on experience.
How does Spring manage Beans differently from other Java objects?
Understanding the difference between Spring Beans and Java Beans is crucial for your candidate to be able to explain the abstractions that Spring builds on top of Java. To hire a great Spring developer, you want a strong Java engineer who understands the Spring Framework instead of engineers who can only write applications with Spring Framework.
Spring manages the lifecycle (instantiation, invocation, destructor) of Spring Beans through the IoC container.
It’s important that your candidate doesn’t get Spring Beans mixed up with Java Beans. Java Beans are a concept outside of the scope of Spring and simply define some interfaces that a Java class must-have. A Spring Bean could be a Java Bean if its lifecycle is managed by Spring.
Check out our entire set of software development interview questions to help you hire the best developers you possibly can.
If you’re a developer, familiarize yourself with the non-technical interview questions commonly asked in the first round by HR recruiters and the questions to ask your interviewer!
Arc is the radically different remote job search platform for developers where companies apply to you. We’ll feature you to great global startups and tech companies hiring remotely so you can land a great remote job in 14 days. We make it easier than ever for software developers and engineers to find great remote jobs. Sign up today and get started.
What are the Bean scopes available in Spring?
As of Spring 5, Spring provides the following Bean scopes: singleton, prototype, request, session, application, and websocket.
If you have created Spring Beans before but did not specify their Bean scopes, then you have been using the singleton scope. Spring will default to using the singleton scope, meaning that one instance of the object will be instantiated during the application’s lifecycle.
Being able to communicate why different Bean scopes should be used can help convey your candidate’s understanding of object lifecycles. Explaining why they chose a specific Bean scope proves that they understand Spring Beans and can make design considerations while producing code. Look for concrete examples of Beans that they have created in the past and why a specific Bean scope was used.
What is the difference between BeanFactory
and ApplicationContext
?
Both BeanFactory
and ApplicationContext
are IoC containers used to manage bean life cycles. The difference is that a BeanFactory
enables a Bean to be lazy-loaded while ApplicationContext
loads beans at application startup.
Spring recommends that you use ApplicationContext
instead of BeanFactory
unless your application requires memory optimization.
Less-experienced developers would potentially be more eager to use BeanFactory
, as it is more performant. A high-quality, experienced developer should be able to explain the design tradeoffs and rationale for why they might choose one over the other.
What is the Bean life cycle in Spring Bean Factory Container?
A Spring Bean is instantiated, its properties are populated, its custom init()
method is called, and then ready to be used. Once it’s to be shut down, it calls its destroy()
method for cleanup.
You may ask about this to gauge your candidate’s understanding of how Spring manages the overhead of Bean instantiation and destruction and when their custom code is invoked during this process.
When is autowiring used?
Autowiring is used to reduce the amount of user-defined configurations by allowing Spring to make implicit decisions on how to manage dependency injection.
There are several ways to achieve the autowiring, but it’s often used with the @Autowired
annotation for field, setter, and constructor injection.
@Autowired
is commonly used, and many Spring tutorials have developers follow along to set the annotations in their code without a good understanding of what it’s doing. Explaining how @Autowired
is used and how Spring uses this to achieve dependency injection allows your candidates to show their depth of Spring knowledge.
What is autowiring? Can you name the different modes of autowiring?
Autowiring is a way to set up dependency injection by relying on Spring to make injections and thereby reducing configurations defined in the application. Five types of autowiring are supported as of Spring 5: no
, byName
, byType
, constructor
, and autodetect
. Autowiring with autodetect
provides too much implicit setup so is being deprecated by Spring.
If you use the @Autowired
annotation and aren’t familiar with the types of autowiring, it’s because @Autowired
automatically chooses the corresponding type depending on where it is defined. If you annotate a property or property setter, the byType
mode is used.
If you annotate a constructor, the constructor
type is mode. Therefore, this question is often used by interviewers to gauge whether an interviewee understands the underlying configurations that Spring annotations can manage on your behalf.
Read More: How to Be More Productive Working at Home
Spring Boot Interview Questions
What is the distinction between Spring Boot and Spring?
Spring Boot is a framework that is an abstraction on top of Spring. It enables developers to efficiently build applications through an opinionated approach. It provides tooling and greatly reduces boilerplate configurations that a developer would typically need in order to build a web application.
Since Spring Boot has reduced a lot of the overhead of setting up configurations, many developers choose it to create backend webservices for RESTful APIs.
Since Spring Boot is very popular, there are many developers who have built projects with Spring Boot but not with Spring. An inexperienced engineer who has only used Spring Boot may force Spring Boot’s abstractions onto a problem when Spring is a better solution. As the interviewer, you’d want to hire an engineer who can explain when it may be appropriate to use Spring over Spring Boot.
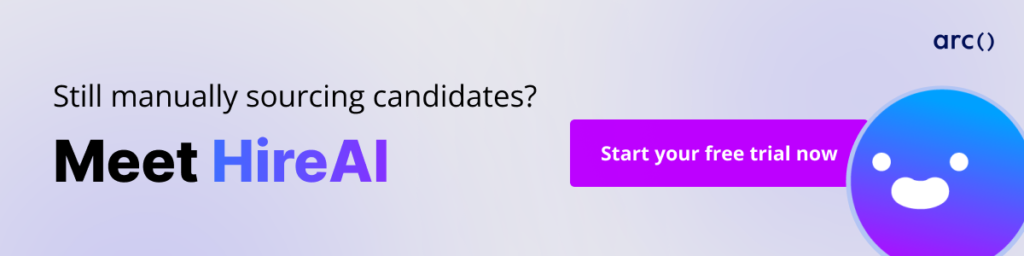
Spring MVC Interview Questions
What is the distinction between Spring MVC and Spring?
Spring MVC (Model-View-Controller) is a library in the Spring Framework that is often used to build Java web applications through the Model-View-Controller approach. In short, Spring brings the ability to set up IoC with dependency injection, and Spring MVC uses that functionality to provide a framework for building web applications.
It’s important that your candidate doesn’t get Spring MVC confused with Spring Boot. Spring MVC is configuration-heavy but allows you feature-rich customizations, whereas Spring Boot is very opinionated with the goal of reducing the overhead of having a developer create a productive web application. They can achieve the same results but with varying time spent on development and configuration.
Since Spring MVC and Spring Boot can achieve the same functionality, it’s important that your candidate can distinguish why they may choose to use one over the other.
What is DispatcherServlet in Spring MVC?
The DispatcherServlet streamlines and handles all of the HTTP requests and responses in Spring MVC. It serves as a front controller and delegates all web requests to corresponding controllers for downstream processing.
Instead of specifically asking about the DispatcherServlet, you may ask your candidate to describe the workflow for how an HTTP request goes through Spring. When asked this, it is important that your candidate indicates that all the requests and responses are funneled through the DispatcherServlet.
What is the ViewResolver pattern? Can you explain its significance in Spring MVC?
The ViewResolver pattern manages the different types of view technologies that can be used to render the view. This abstraction makes view technologies configuration-based, enabling decoupling from view technologies such as JSP and XSLT.
This aligns with a common software pattern known as the separation of concerns. A developer can be focused on writing view content. The view resolver will handle the details of how the view content gets processed into different view technologies.
Since view resolvers are for rendering views, it’s important to note that RESTful applications typically do not need them. RESTful applications simply return HTTP responses. Therefore, when you ask your candidate to design a RESTful API using Spring MVC, they should remember to forgo including the view resolver in the design details.
What is a Controller in Spring MVC?
A Spring MVC Controller is a class that’s used to handle web requests. It typically contains the business logic of an application.
Controllers in MVC patterns often manage the “how” of your application code. Usually, your controller logic will involve how you handle the various fields in an inbound HTTP request and how it’s processed into an HTTP response.
You may ask your candidate to use Spring MVC to set up a RESTful application. In these scenarios, you want to see if they choose to use a RestController
in lieu of a Spring Controller. A RestController
will send raw data as an HTTP response and help simplify their RESTful application.
How does the @Requestmapping
annotation Work?
The @RequestMapping
annotation defines the method that handles the specified HTTP request. @RequestMapping
can generally be configured with values, headers, and request types.
For example, if we have the following request mapping annotating a method:
@RequestMapping(value = "/transactions", method = GET)
This specifies that the method will handle the HTTP GET
requests that are made to the /transactions
endpoint.
You may ask your candidate to work on a design exercise to map out the requests and responses that an API will handle. This will involve deciding what type of endpoints to create and their corresponding HTTP methods. They may be able to use the @RequestMapping
syntax to convey their API design choices.
Spring AOP, Spring JDBC & Spring Hibernate
What is Spring Aspect-Oriented Programming (AOP)?
Spring Aspect-oriented programming (AOP) enables an application to be designed with cross-cutting logic between modules. Spring provides interfaces such as annotations to implement AOP functionality. Spring AOP is configured with an aspect that defines a concern, a joinpoint for when to execute the logic, and a pointcut that applies a specific action known as an advice.
One common example is how an application handles logging. Logging should affect the entire system and be specific to one module in the application. Spring AOP defines a pattern for how logging logic can be defined and integrated into the rest of the application.
You may not explicitly ask your candidate what Spring AOP is for. Instead, you may ask them to explain how they’d design or architect different business requirements into a Spring application. By identifying business functionality that affects other modules, they may be able to propose using Spring AOP to organize their application architecture.
What is an advice? Explain its types in Spring.
An advice is an action that is applied at a specific point of a program’s execution. It can be configured as a before
type to run prior to method execution, an after
type to run after method execution, and an around
type to run before and after method execution.
This provides a powerful pattern for modifying a method’s behavior. For example, imagine that you wanted to understand the execution time for all of your methods. If you were to implement this functionality yourself, you could use the before
and after
types to cleanly track when the method is about to be invoked and when it finishes.
Knowing how and when to use an advice is a strong signal to the interviewer of your understanding of design principles with Spring. Even if you don’t explicitly ask about advice, you may want to look for developers who are able to offer it as a solution for modeling cross-functional business logic.
What are some of the classes for Spring JDBC API?
One of the core classes in Spring JDBC is the JdbcTemplate
class. It handles the overhead of the database connection and exposes an interface to query a relational database. RowMapper
and ResultSetExtractor
are two classes that are commonly used to handle the data that is returned from a database query.
You may ask this question to gauge your candidate’s familiarity with the Spring JDBC API. The goal isn’t to see if they have memorized every class of the Spring JDBC API. Instead, your goal is to set the stage for your candidate to recall a class and explain the context of when they used it.
What are the tradeoffs for using JdbcTemplate
versus Jdbc
?
JdbcTemplate
is a thin wrapper built on top of Jdbc
that removes boilerplate configuration and management of the database connection to make development simpler in Spring. While often unnecessary, one could possibly explore profiling the performance penalty of using JdbcTemplate
versus Jdbc
when processing large volumes of data due to JdbcTemplate
’s overhead.
You may ask this question to gauge whether your candidate follows best practices and uses JdbcTemplate
. If they have claimed to have used Jdbc
, you may follow up and ask them about their decision to opt out of using JdbcTemplate
to validate their design decisions in doing so.
Wrapping Up
Technical interviews aren’t easy, that’s for sure.
Technical interviews aren’t easy, that’s for sure. Keep in mind, while your technical interview’s main purpose is to assess your candidate’s expertise in the framework, how they frame their answers is just as important as what they say.
When interviewing a Spring developer, make sure to help them draw out their thought process. By setting the stage for your candidate, you can better understand their problem-solving skills and communication skills. Remember, soft skills are just as important as technical skills, so make sure to gauge your candidate’s skills holistically.
We wish you the best of luck in hiring a Spring developers!
You can also explore HireAI to skip the line and:
⚡️ Get instant candidate matches without searching
⚡️ Identify top applicants from our network of 250,000+ devs with no manual screening
⚡️ Hire 4x faster with vetted candidates (qualified and interview-ready)
great piece of content
well written article