MongoDB is one of the latest and greatest competitors in data storage. This document-oriented database platform has gained tremendous popularity when compared to traditional relational database management systems (RDBMS).
Using MongoDB requires complex coding skills and knowledge. When you’re looking to hire a developer who uses MongoDB, whether it’s a database administrator or a back-end developer, your interview questions should test their technical skills as well as problem solving skills.
The interview questions in this article will help cover many of the questions that are commonly asked in a MongoDB interview. Mix and match the interview questions below to fit your MongoDB developer job descriptions.
- Basic MongoDB Interview Questions
- Intermediate MongoDB Interview Questions
- Advanced MongoDB Interview Questions
- More Questions to Understand for MongoDB Interviews
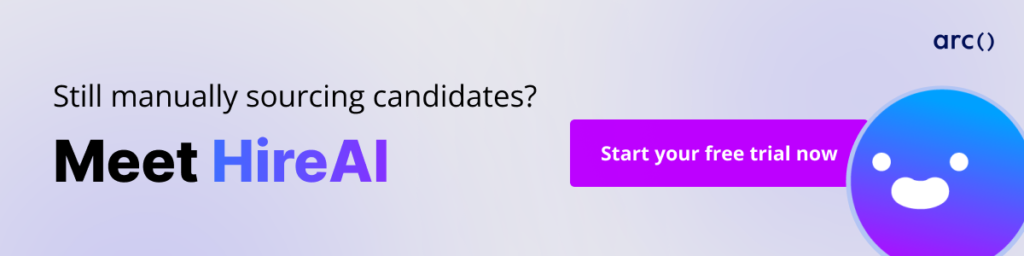
Basic MongoDB Interview Questions
1. Compare MongoDB With SQL Server
SQL Server is an open-source RDBMS where you store data in tables and rows and query using a structured query language (SQL). When users need this data, they must construct an SQL query that joins multiple tables together to fetch the result.
However, MongoDB is also open-source and its design principles vary; they are styled as a non-relational (NoSQL) system. As opposed to tables and row format, they store data in JSON-like documents.
You might ask this question to test your candidates’ fundamental understanding of SQL databases’ common characteristics before asking questions on NoSQL databases.
Here are some of the head-to-head differences between MongoDB and SQL Server you should look for in your candidate’s answer:
SQL Server | MongoDB |
---|---|
The database model used here is RDBMS | The database model used is the document store |
Written in C and C++ | Written in C, C++, Go, JavaScript, and Python languages |
SQL server support XML data format | MongoDB does not support the XML data format |
The data schema is fixed | The data schema is dynamic |
Has vertical scalability | Has horizontal scalability |
Uses joins | Does not use joins |
Uses ACID transaction properties | Does not use ACID properties |
Does not support map-reduce method | Supports map-reduce method |
The license of SQL Server is commercial | Open source license |
Uses concepts like referential integrity and foreign keys | There is no foreign keys concept here. |
2. What Are Other Types of NoSQL Databases?
As an interviewer, you would expect a good candidate to know why they’d want to use a NoSQL database, what the purpose of using it is, and so forth. They should also be aware of other NoSQL databases apart from MongoDB, and when to use other NoSQL databases.
Encourage your candidate to provide examples or scenarios of when they might use other types of NoSQL databases. Here are some other NoSQL databases:
- Document databases – Stores data in documents (XML, JSON, YAML, BSON, etc.). Examples of document databases include: Amazon SimpleDB, Apache CouchDB, MongoDB, and Couchbase Server.
- Key-Value (KV) stores – The simplest database type, a key-value database stores data in pairs (attributes, or “keys,” and “values”). Examples of key-value databases include: Apache Ignite, Riak, and Amazon’s DynamoDB.
- Column-oriented databases – These are NoSQL databases which store data in columns, and they are widely used to manage data warehouses, business intelligence, CRMs, library card catalogs, etc. Examples of columnar databases include: Apache Cassandra and Apache HBase.
- Graph databases – Graph databases are mostly used to find connections between data elements. Examples of graph databases include: ArangoDB, Amazon Neptune, Neo4j, and Stardog.
If you need more detailed information, you can check out the differences between the four types of NoSQL databases.
Read More: How to Write a Great Thank-You Email After an Interview
3. What Is a Document in MongoDB?
A document is like a unit where you can store your MongoDB data (JSON-styled). This document will again contain field names and values.
For example, in the below simple example of a JSON document, the site is a “field” and arc.dev is a “value”.
{ site: “arc.dev” } //field: value
You want your candidate to know about MongoDB’s _id
field and how to use them. This is because all documents in MongoDB must have a populated _id
field. If not, MongoDB will generate one for you.
Also, you want to ensure your candidate understands the flexibility that MongoDB provides in its document-based design, which allows developers to define multiple BSON data types as field values. This is a feature that contrasts with other relational databases.
You can ask the candidate to show a small and basic example of a document in MongoDB.
var mydoc = {
_id: ObjectId(“353329803df3f4948bd2345fd391”),
name: { first: “John”, last: “Doe” },
birth: new Date(‘Apr 12, 1912’),
death: new Date(‘Jun 07, 1954’),
contribs: [ “Turing machine”, “Turing test”],
views : NumberLong(1250000)
}
When you group multiple documents into a structure, it is called a collection. You can now ask questions based on collections in MongoDB.
4. How Do You Create a Collection in MongoDB?
The basic step in any MongoDB application is used to create a database and a collection. Because the database stores the information about the collection and the collection in turn stores all the documents.
So when you ask about a collection, your candidate must know how to create a database using the use
command and create a collection using the createCollection()
method. They must also know the different ways to create a collection.
To best answer this question, they’d ideal show how to create a collection using the two methods below:
Method 1: On the fly
In a single line of command, you can insert a document into the collection. MongoDB creates a collection for you on the fly.
Syntax: db.collection_name.insert({key:value, key:value…})
Method 2: Create options before inserting the documents
When you create a collection before you insert the data, you can have the flexibility to set it while creating a collection.
Syntax: db.createCollection(name, options)
This activity allows you to gauge whether your candidate can create a collection in MongoDB. This is one of the most expected questions in MongoDB interviews, as this is the first and foremost step after MongoDB database has been created.
Read More: Phone Screen Interview vs Actual Phone Interview: Learn the Differences
Intermediate MongoDB Interview Questions
1. How to Create Queries in MongoDB?
By this time, you should have covered most of the basic questions on databases and collections in MongoDB. The next part should cover filtering and applying conditions in MongoDB queries.
You might ask this question to find out the different methods that your candidate uses in their project applications while applying filtering to MongoDB queries. The best answers should include some of the commonly used query mechanisms that MongoDB provides when filtering and fetching specific documents.
You can ask the candidate a few syntaxes of the filtering methods to filter the results in MongoDB.
find()
method: Using this method you can query the data from the MongoDB collection.
Syntax: db.COLLECTION_NAME.find()
pretty()
method: Used to pretty format the results displayed.
Syntax: db.COLLECTION_NAME.find().pretty()
findOne()
method: Returns only one document.
Syntax: db.COLLECTIONNAME.findOne()
You may also gauge your candidates’ skills by asking them some more complex queries containing different fields of different types, and also to fetch a document within a nested document structure.
For more examples, refer to the Query documents page from MongoDB docs.
2. What Are Indexes in MongoDB?
When you query a MongoDB database by using specific conditions to retrieve documents, the database performs a scan in your collection to retrieve the result. i.e., it will retrieve every document from the collection to verify whether they match the condition. If there is a match, it will be added to the list of returned documents. If not, MongoDB scans the next document until it has scanned the entire collection.
When you ask this question, you are looking for how well the interviewee knows about the impact of the indexes, how indexes work, and how they are implemented in MongoDB.
The best answer about indexes’ impact should include an example of the products in an eCommerce store, where each product is represented by a document containing images, descriptions, prices, and more. Without indexes, MongoDB retrieves all products from the collection. With an index, MongoDB can use a smaller list containing pointers to only products in stock.
Here, one of the key points that your candidate must address in their answer about Indexes is that they are inclusive, meaning MongoDB knows how to answer them even when you include only documents in the indexes. To demonstrate this explanation, they can use the below example.
{
Empid: 1
Empcode: ABC
Empname: “John”
Country: “India”
}
As you can see, the Empid
and Empcode
are indexes in this collection. So when you create a query, these indexes fetch the required document quickly. You can refer to more indexing strategies from the official MongoDB documentation.
Read More: 10+ Tips for Preparing for a Remote Software Developer Zoom Interview
3. What Are Geospatial Indexes in MongoDB?
As a technical interviewer, you may ask this question to see if your candidate’s familiar with the different types of queries they can use to execute on a collection containing geospatial shapes and points. Their answer should include both types of geospatial indexes in MongoDB.
There are two geospatial indexes in MongoDB: 2dsphere
and 2d
. If you are working with spherical geometries based on the WGS84 datum, then you can use 2dsphere
. In this WGS84 datum model, there is some flattening at the poles near the surface of the earth. You can also specify geometries for lines, points, and polygons in the GeoJSON format.
Your candidate might use the following simple example to demonstrate the geospatial indexes in MongoDB.
{
“name”: “New York City”,
“loc” : {
“type” : “Point”,
“coordinates” : [50, 2]
}
}
If you’re looking for a MongoDB developer who has experience working with maps or navigation, you’d want to know how your candidate would implement the application and what spatial queries they’ve used on a collection containing geospatial points.
4. What Is Replication and Why Do You Use It?
Replication means synchronizing data across multiple servers, which increases data availability. In case you lose a single server, then your data is still intact and protected by being stored on other servers.
Here, you want to gauge the candidate’s understanding of the concept of replication. A good answer should cover how replication may help in their application or how they’d protect the database upon loss of a single server or a hardware failure. On top of that, you may want to ask about past application infrastructure they’ve worked with and whether they’ve used multiple servers with data replication before.
How does replication work?
MongoDB uses replica sets to achieve replication. The replica sets are a collection of different MongoDB instances that can act as hosts to the datasets. There are ideally two types of replica sets:
Primary replica set – This is the master replica set to which MongoDB writes data (receives all write operations).
Secondary replica set – This is a replica of the primary replica set’s oplog (operations log, records all operations that modify data in the replica set).
To study up more on deploying replica sets before your interview, you can refer to the detailed documentation from MongoDB.
Read More: 8 Behavioral Interview Questions Asked by Top Tech Companies
5. What Are Embedded Documents in MongoDB?
Embedded documents in MongoDB allow you to store all kinds of information related to each other in a single document. An embedded (nested) MongoDB Document is a normal document that resides inside another document within a MongoDB collection.
You’d likely ask this question to understand if your candidate has a good grasp of when to use embedded documents in MongoDB. In a technical interview, ask your candidate to write code to explain how to use embedded MongoDB documents.
The following are a few general guidelines of common scenarios which call for using embedded documents:
- When your application is using a one-to-few relationship
- When updates to your document are likely to happen at the same time
- When the field is rarely updated
Some key points to look for in an answer about embedded documents include:
- In MongoDB, the size of the document must be smaller than the maximum BSON document size (16MB).
- If you delete the parent MongoDB document, then child MongoDB documents are also deleted.
Looking to hire the best remote developers? Explore HireAI to see how you can:
⚡️ Get instant candidate matches without searching
⚡️ Identify top applicants from our network of 300,000+ devs with no manual screening
⚡️ Hire 4x faster with vetted candidates (qualified and interview-ready)
Try HireAI and hire top developers now →
Advanced MongoDB Interview Questions
1. What Is the Role of the Database Profiler in MongoDB?
The profiler can be used as a debugging tool to analyze slow-performing queries or transactions. Getting a profile for this performance can greatly help software developers identify bottlenecks and misconfigurations.
The MongoDB profiler collects comprehensive data about database commands executed, write operations, cursors, etc. against a running mongod
instance.
As the interviewer, you’d want to hear about how he or she, as a developer, can tackle slow-performing queries and performance issues in MongoDB.
To answer this question, your candidate must know the different levels in the MongoDB profiler and how they can enable or configure them. See database profiler for more details.
Importantly, make sure they understand the profiling levels that you may ask.
Level | Description |
---|---|
0 | The profiler is off by default and cannot log any data. |
1 | The profiler will log only slow operations above some threshold. |
2 | The profiler will log all the operations. |
Read More: 8 Common Interview Mistakes Remote Software Developers Make
2. What Is Sharding in MongoDB?
Sharding is the process MongoDB uses to distribute data across multiple hosts.
When you are developing complex applications with large amounts of data within MongoDB, it can impact CPU utilization. The solution is to split these large data sets into small data sets across different MongoDB instances, which can be achieved by a concept in MongoDB called sharding.
When you ask this question, you want to see how well your candidate knows the concept of sharding, the way it works, and what would happen if sharding not implemented. To best answer this question, learn more about Sharding from the MongoDB documentation.
3. What Is Map-Reduce in MongoDB?
You may ask this question to gauge your candidate’s understanding of different data processing techniques when they use large data in their applications.
To answer this question, your candidate must first understand why the map function is called a mapper (since it creates a map of data in the form of key-value pair) and why the reduce function is called a reducer (since it aggregates the data that the mapper has generated).
Map-reduce commands in MongoDB are made up of two higher-order functions: map()
and reduce()
. From the name, it implies that the map()
job is performed before the reduce()
job.
Read More: 35 Questions to Ask at an Interview for Software Development Jobs
4. How Can We Sort the User-Defined Function?
For example, if x and y are integers, how do we calculate “x-y”?
The purpose of these interview questions is to see if your candidate understands the concept of the aggregation pipeline in MongoDB used to aggregate tasks. The pipeline transforms the documents into aggregated results.
So, to calculate x-y, we use the below function:
db.eval(function() {
return db.scratch.find().toArray().sort(function(arg1, arg22) {
return arg1.a – arg2.a
})
});
//The equivalent client-side sort:
db.scratch.find().toArray().sort(function(arg1, arg2) {
return arg1.a – arg2.b
});
5. What Is a Covered Query in MongoDB?
A covered query is one that uses an index and doesn’t require the examination of any documents. You’d ask this question to test your candidate’s knowledge of the differences between using ordinary queries vs. covered queries.
More MongoDB Interview Questions to Practice
Before we wrap this article up, here are a few other MongoDB interview questions you might want to ask. To screen your candidates more holistically, don’t ignore these, no matter how basic they might seem:
- Which RDBMS would you choose if you want to perform quick schema changes and can be optimized for speed?
- Can journaling features be used to perform safe hot backups?
- Does the MongoDB database have tables for storing records?
- Do the MongoDB databases have a schema?
- By default, which replica sets are used to write data?
- Is it possible to configure the cache size for MMAPv1 in MongoDB?
- Which language is used to write for MongoDB?
Read More: How to Answer Non-Technical Interview Questions
Conclusion
This article has covered some of the most frequently asked MongoDB interview questions and answers. But, besides knowing the questions and answers, your perfect candidate should ideally understand the MongoDB environment, how to optimize and improve performance in MongoDB, and how to perform more advanced applications using MongoDB.
Here are a few further references to understand MongoDB in-depth:
You can also explore HireAI to skip the line and:
⚡️ Get instant candidate matches without searching
⚡️ Identify top applicants from our network of 250,000+ devs with no manual screening
⚡️ Hire 4x faster with vetted candidates (qualified and interview-ready)